Métodos más Comunes.
Drag Inicia, termina o cancela una operación de arrastre de cualquier control, excepto los controles Line, Menu, Shape, Timer o CommonDialog.
Move Se utiliza para mover un control o formulario, especificando sus coordenadas (Top, Left) y su tamaño (Width, Height).
Refresh Se utiliza para dibujar o actualizar gráficamente un control o un formulario. Se utiliza principalmente con los controles FileListBox y Data.
SetFocus Este método se utiliza para hacer que un objeto reciba el enfoque. Este método es uno de los más usados para los controles de Visual Basic 6.0.
ShowWhatsThis Permite mostrar un tema seleccionado de un archivo de Ayuda utilizando el menú emergente ¿Qué es esto? que ofrece la ayuda de Windows. Este método es muy útil para proporcionar ayuda interactiva en un menú contextual acerca de un objeto en una aplicación. Este método muestra el tema indicado por la propiedad WhatsThisHelpID del objeto especificado en la sintaxis.
Zorder Se utiliza para que un control o un objeto formulario se coloque por encima o por debajo de otros objetos.
PROPIEDADES DE VISUAL BASIC
PROPIEDADES COMUNES DE LOS CONTROLES
Los controles que se pueden incluir en el formulario aparecen en la caja de herramientas. No es necesario recordar la posición o forma de cada control. En Visual Basic 4.0, cuando el cursor se sitúa sobre un control de la caja de herramientas, aparece una pista recordando el control que permite crear. Todos los controles tienen algunas propiedades comunes como pueden ser:
Caption (Leyenda)
Establece el texto que el usuario visualizará. No confundir con la propiedad Name.
Text (Texto)
Actúa igual que la propiedad Caption para aquellos controles que no dispongan de dicha propiedad, p.e. las cajas de texto. Muestra el contenido del control y, por tanto, también contiene los caracteres introducidos por el usuario. No confundir con la propiedad
Name.
Name (Nombre)
Propiedad MUY importante. Define el nombre del control en el código del programa. No confundir con las propiedades Caption o
Text que es lo que el usuario visualiza.
TabStop (Punto de Tabulación)
Si el valor es True, el control será susceptible de recibir el foco durante la ejecución de la aplicación.
TabIndex (Índice de tabulación)
Indica el número de orden en el que el control recibirá el foco cuando el usuario, en tiempo de ejecución, pulse la tecla Tab para recorrer los controles. A medida que se van situando controles en el formulario, Visual Basic incrementa en una unidad, el valor de esta propiedad para el nuevo control y, lo decrementa en caso de eliminar algún control. El valor para el primer control es 0.
PROPIEDADES DE LOS BOTONES DE COMANDO
Command Button (2ª fila, icono de la derecha). Se utiliza para ejecutar la acción asociada a la pulsación de dicho botón.
Enabled (Habilitado)
El valor False hace que el botón aparezca atenuado y, no responda a eventos.
Cancel (Cancelar)
Establecer el valor de esta propiedad a True, hace que el botón responda a la pulsación de la tecla ESC como si se hubiera hecho clic sobre él. En un...
Esta página está creada con la intención de hacer conciencia y que por medio de el hagamos saber todas las atrocidades que se cometen a diario con los animales que tenga cabida toda clase de denuncias relacionadas con el maltrato animal de cualquier forma, y de una manera u otra terminar con esta clase prácticas o para algunos " deporte" tan terribles
lunes, 16 de mayo de 2011
lunes, 9 de mayo de 2011
lunes, 2 de mayo de 2011
IF-THEN-ELSE IF-END IF
IF-THEN-ELSE IF-END IF
The Nested IF-THEN-ELSE-END IF statement could produce a deeply nested IF statement which is difficult to read. There is a short hand to overcome this problem. It is the IF-THEN-ELSE IF-END-IF version. Its syntax is shown below:
IF (logical-expression-1) THEN
statements-1
ELSE IF (logical-expression-2) THEN
statements-2
ELSE IF (logical-expression-3) THEN
statement-3
ELSE IF (.....) THEN
...........
ELSE
statements-ELSE
END IF
Fortran evaluates logical-expression-1 and if the result is .TRUE., statements-1 is executed followed by the statement after END IF. If logical-expression-1 is .FALSE., Fortran evaluates logical-expression-2 and executes statements-2 and so on. In general, if logical-expression-n is .TRUE., statements-n is executed followed by the statement after END IF; otherwise, Fortran continues to evaluate the next logical expression.
If all logical expressions are .FALSE. and if ELSE is there, Fortran executes the statements-ELSE; otherwise, Fortran executes the statement after the END IF.
Note that the statements in the THEN section, ELSE IF section, and ELSE section can be another IF statement.
Examples
Suppose we need a program segment to read a number x and display its sign. More precisely, if x is positive, a + is displayed; if x is negative, a - is displayed; otherwise, a 0 is displayed. Here is a possible solution using IF-THEN-ELSE IF-END IF:
IF (x > 0) THEN
WRITE(*,*) '+'
ELSE IF (x == 0) THEN
WRITE(*,*) '0'
ELSE
WRITE(*,*) '-'
END IF
Given a x, we want to display the value of -x if x < 0, the value of x*x if x is in the range of 0 and 1 inclusive, and the value of 2*x if x is greater than 1.
The following is a possible solution:
IF (x < 0) THEN
WRITE(*,*) -x
ELSE IF (x <= 1) THEN
WRITE(*,*) x*x
ELSE
WRITE(*,*) 2*x
END IF
Consider the following code segment:
INTEGER :: x
CHARACTER(LEN=1) :: Grade
IF (x < 50) THEN
Grade = 'F'
ELSE IF (x < 60) THEN
Grade = 'D'
ELSE IF (x < 70) THEN
Grade = 'C'
ELSE IF (x < 80) THEN
Grade = 'B'
ELSE
Grade = 'A'
END IF
The Nested IF-THEN-ELSE-END IF statement could produce a deeply nested IF statement which is difficult to read. There is a short hand to overcome this problem. It is the IF-THEN-ELSE IF-END-IF version. Its syntax is shown below:
IF (logical-expression-1) THEN
statements-1
ELSE IF (logical-expression-2) THEN
statements-2
ELSE IF (logical-expression-3) THEN
statement-3
ELSE IF (.....) THEN
...........
ELSE
statements-ELSE
END IF
Fortran evaluates logical-expression-1 and if the result is .TRUE., statements-1 is executed followed by the statement after END IF. If logical-expression-1 is .FALSE., Fortran evaluates logical-expression-2 and executes statements-2 and so on. In general, if logical-expression-n is .TRUE., statements-n is executed followed by the statement after END IF; otherwise, Fortran continues to evaluate the next logical expression.
If all logical expressions are .FALSE. and if ELSE is there, Fortran executes the statements-ELSE; otherwise, Fortran executes the statement after the END IF.
Note that the statements in the THEN section, ELSE IF section, and ELSE section can be another IF statement.
Examples
Suppose we need a program segment to read a number x and display its sign. More precisely, if x is positive, a + is displayed; if x is negative, a - is displayed; otherwise, a 0 is displayed. Here is a possible solution using IF-THEN-ELSE IF-END IF:
IF (x > 0) THEN
WRITE(*,*) '+'
ELSE IF (x == 0) THEN
WRITE(*,*) '0'
ELSE
WRITE(*,*) '-'
END IF
Given a x, we want to display the value of -x if x < 0, the value of x*x if x is in the range of 0 and 1 inclusive, and the value of 2*x if x is greater than 1.
The following is a possible solution:
IF (x < 0) THEN
WRITE(*,*) -x
ELSE IF (x <= 1) THEN
WRITE(*,*) x*x
ELSE
WRITE(*,*) 2*x
END IF
Consider the following code segment:
INTEGER :: x
CHARACTER(LEN=1) :: Grade
IF (x < 50) THEN
Grade = 'F'
ELSE IF (x < 60) THEN
Grade = 'D'
ELSE IF (x < 70) THEN
Grade = 'C'
ELSE IF (x < 80) THEN
Grade = 'B'
ELSE
Grade = 'A'
END IF
IF-THEN-END
The IF-THEN-END IF form is a simplification of the general IF-THEN-ELSE-END IF form with the ELSE part omitted:
IF (logical-expression) THEN
statements
END IF
where statements is a sequence of executable statements, and logical-expression is a logical expression. The execution of this IF-THEN-ELSE-END IF statement goes as follows:
the logical-expression is evaluated, yielding a logical value
if the result is .TRUE., the statements in statements are executed, followed by the statement following the IF-THEN-END IF statement.
if the result is .FALSE., the statement following the IF-THEN-END IF is executed. In other words, if logical-expression is .FALSE., there is no action taken.
Examples
The following program segment computes the absolute value of X and saves the result into variable Absolute_X. Recall that the absolute value of x is x if x is non-negative; otherwise, the absolute value is -x. For example, the absolute value of 5 is 5 and the absolute value of -4 is 4=-(-4). Also note that the WRITE(*,*) statement has been intentionally broken into two lines with the continuation line symbol &. The trick is that the value of X is first saved to Absolute_X whose value is changed later only if the value of X is less than zero.
REAL :: X, Absolute_X
X = .....
Absolute_X = X
IF (X < 0.0) THEN
Absolute_X = -X
END IF
WRITE(*,*) 'The absolute value of ', x, &
' is ', Absolute_X
The following program segment reads in two integer values into a and b and finds the smaller one into Smaller. Note that the WRITE(*,*) has also been broken into two lines. This uses the same trick discussed in the previous example.
INTEGER :: a, b, Smaller
READ(*,*) a, b
Smaller = a
IF (a > b) THEN
Smaller = b
END IF
Write(*,*) 'The smaller of ', a, ' and ', &
b, ' is ', Smaller
IF (logical-expression) THEN
statements
END IF
where statements is a sequence of executable statements, and logical-expression is a logical expression. The execution of this IF-THEN-ELSE-END IF statement goes as follows:
the logical-expression is evaluated, yielding a logical value
if the result is .TRUE., the statements in statements are executed, followed by the statement following the IF-THEN-END IF statement.
if the result is .FALSE., the statement following the IF-THEN-END IF is executed. In other words, if logical-expression is .FALSE., there is no action taken.
Examples
The following program segment computes the absolute value of X and saves the result into variable Absolute_X. Recall that the absolute value of x is x if x is non-negative; otherwise, the absolute value is -x. For example, the absolute value of 5 is 5 and the absolute value of -4 is 4=-(-4). Also note that the WRITE(*,*) statement has been intentionally broken into two lines with the continuation line symbol &. The trick is that the value of X is first saved to Absolute_X whose value is changed later only if the value of X is less than zero.
REAL :: X, Absolute_X
X = .....
Absolute_X = X
IF (X < 0.0) THEN
Absolute_X = -X
END IF
WRITE(*,*) 'The absolute value of ', x, &
' is ', Absolute_X
The following program segment reads in two integer values into a and b and finds the smaller one into Smaller. Note that the WRITE(*,*) has also been broken into two lines. This uses the same trick discussed in the previous example.
INTEGER :: a, b, Smaller
READ(*,*) a, b
Smaller = a
IF (a > b) THEN
Smaller = b
END IF
Write(*,*) 'The smaller of ', a, ' and ', &
b, ' is ', Smaller
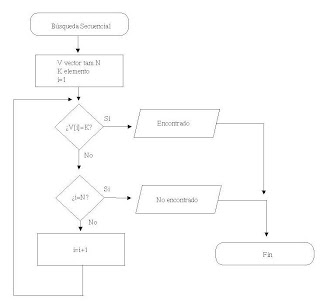
algotimo
ALGORITMO
En resumen, un algoritmo es cualquier cosa que funcione paso a paso, donde cada paso se pueda describir sin ambigüedad y sin hacer referencia a una computadora en particular, y además tiene un límite fijo en cuanto a la cantidad de datos que se pueden leer/escribir en un solo paso. Esta amplia definición abarca tanto a algoritmos prácticos como aquellos que solo funcionan en teoría, por ejemplo el método de Newton y la eliminación de Gauss-Jordan funcionan, al menos en principio, con números de precisión infinita; sin embargo no es posible programar la precisión infinita en una computadora, y no por ello dejan de ser algoritmos.[10] En particular es posible considerar una cuarta propiedad que puede ser usada para validar la tesis de Church-Turing de que toda función calculable se puede programar en una máquina de Turing (o equivalentemente, en un lenguaje de programación suficientemente general):[10]
Suscribirse a:
Entradas (Atom)